1. Import packages¶
[1]:
import os,sys
%matplotlib inline
import matplotlib.pylab as plt
import numpy as np
plt.rcParams['figure.dpi'] = 100
plt.rcParams['savefig.dpi']=300
plt.rcParams['font.family']='sans serif' #please remove this line if font is not installed
plt.rcParams['font.sans-serif']='Arial' # please remove this line if Arial font is not installed
plt.rcParams['pdf.fonttype']=42
# sys.path.append(os.path.expanduser("~/Projects/Github/PyComplexHeatmap/"))
import PyComplexHeatmap as pch
print(pch.__version__)
1.7.7.dev0+gddb18da.d20240909
2. Plot heatmap annotations¶
[2]:
#Generate example dataset (random)
df = pd.DataFrame(['GroupA'] * 5 + ['GroupB'] * 5, columns=['AB'])
df['CD'] = ['C'] * 3 + ['D'] * 3 + ['G'] * 4
df['EF'] = ['E'] * 6 + ['F'] * 2 + ['H'] * 2
df['F'] = np.random.normal(0, 1, 10)
df.index = ['sample' + str(i) for i in range(1, df.shape[0] + 1)]
df.head()
[2]:
AB | CD | EF | F | |
---|---|---|---|---|
sample1 | GroupA | C | E | -0.709613 |
sample2 | GroupA | C | E | 0.029959 |
sample3 | GroupA | C | E | 1.580365 |
sample4 | GroupA | D | E | -0.228082 |
sample5 | GroupA | D | E | 1.116552 |
[3]:
plt.figure(figsize=(3, 4))
col_ha = pch.HeatmapAnnotation(df=df,plot=True,legend=True,legend_gap=5,hgap=0.5,axis=1)
plt.show()
Plotting HeatmapAnnotations
Collecting annotation legends..
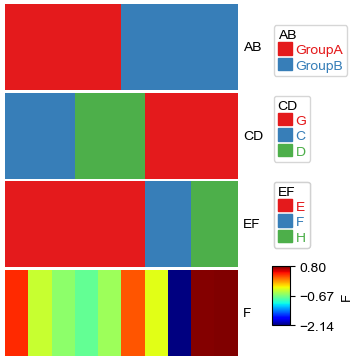
We can choose pass the whole dataframe df
to HeatmapAnnotation, or pass each column of df
to different kinds of annotation function separately.
[4]:
df_box = pd.DataFrame(np.random.randn(10, 4), columns=['Gene' + str(i) for i in range(1, 5)])
df_box.index = ['sample' + str(i) for i in range(1, df_box.shape[0] + 1)]
df_bar = pd.DataFrame(np.random.uniform(0, 10, (10, 2)), columns=['TMB1', 'TMB2'])
df_bar.index = ['sample' + str(i) for i in range(1, df_box.shape[0] + 1)]
df_scatter = pd.DataFrame(np.random.uniform(0, 10, 10), columns=['Scatter'])
df_scatter.index = ['sample' + str(i) for i in range(1, df_box.shape[0] + 1)]
df_bar1 = pd.DataFrame(np.random.uniform(0, 10, (10, 2)), columns=['T1-A', 'T1-B'])
df_bar1.index = ['sample' + str(i) for i in range(1, df_box.shape[0] + 1)]
df_bar4 = pd.DataFrame(np.random.uniform(0, 10, (10, 1)), columns=['T4'])
df_bar4.index = ['sample' + str(i) for i in range(1, df_box.shape[0] + 1)]
df_bar4.iloc[7,0]=np.nan
plt.figure(figsize=(3.5, 5))
col_ha = pch.HeatmapAnnotation(label=pch.anno_label(df.AB, merge=True,rotation=15),
AB=pch.anno_simple(df.AB,add_text=True,legend=True), axis=1,
CD=pch.anno_simple(df.CD, add_text=True,legend=True,text_kws={'color':'black'}),
Exp=pch.anno_boxplot(df_box, cmap='turbo',legend=True),
Scatter=pch.anno_scatterplot(df_scatter,grid=True),
Bar1=pch.anno_barplot(df_bar1,legend=True,cmap='Dark2'),
Bar4=pch.anno_barplot(df_bar4,legend=True,cmap='turbo'),
plot=True,legend=True,legend_gap=5,hgap=0.5)
col_ha.show_ticklabels(df.index.tolist(),fontdict={'color':'blue'},rotation=-30)
plt.show()
Plotting HeatmapAnnotations
Collecting annotation legends..
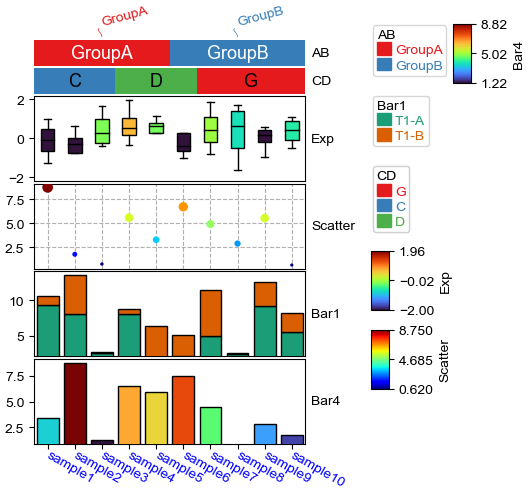
3. Plot the heatmap with rows and columns split¶
[8]:
df_heatmap = pd.DataFrame(np.random.randn(30, 10), columns=['sample' + str(i) for i in range(1, 11)])
df_heatmap.index = ["Fea" + str(i) for i in range(1, df_heatmap.shape[0] + 1)]
df_heatmap.iloc[1, 2] = np.nan
plt.figure(figsize=(3.5, 6))
cm = pch.ClusterMapPlotter(data=df_heatmap,
col_cluster=True,row_cluster=True,
col_split=df.AB,row_split=2,
col_split_gap=0.5,row_split_gap=0.8,
label='values',row_dendrogram=True,
show_rownames=True,show_colnames=True,
row_names_side='right',yticklabels_kws=dict(right=True),
tree_kws={'row_cmap': 'Set1','colors':'blue'},verbose=0,legend_gap=5,
cmap='RdYlBu_r',xticklabels_kws={'labelrotation':-90,'labelcolor':'blue'})
plt.savefig("example0.pdf", bbox_inches='tight')
plt.show()
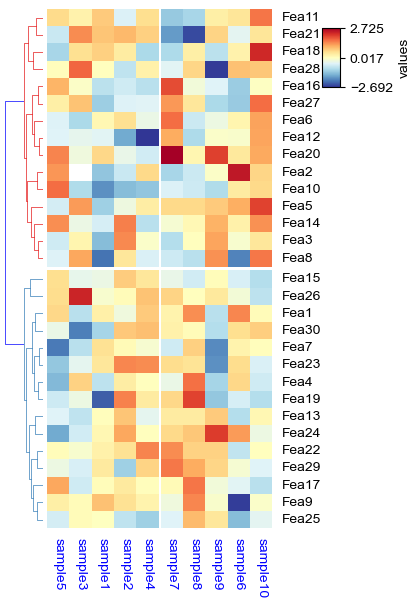
4. Plot the annotations along side with main heatmap¶
[13]:
df_rows = df_heatmap.apply(lambda x:x.name if x.sample4 > 0.5 else None,axis=1)
df_rows=df_rows.to_frame(name='Selected')
df_rows['XY']=df_rows.index.to_series().apply(lambda x:'A' if int(x.replace('Fea',''))>=15 else 'B')
col_ha = pch.HeatmapAnnotation(label=pch.anno_label(df.AB, merge=True,rotation=15),
AB=pch.anno_simple(df.AB,add_text=True,legend=True), axis=1,
CD=pch.anno_simple(df.CD, add_text=True,legend=True,text_kws={'color':'black'}),
Exp=pch.anno_boxplot(df_box, cmap='turbo',legend=True),
Scatter=pch.anno_scatterplot(df_scatter),
Bar1=pch.anno_barplot(df_bar1,legend=True,cmap='Dark2'),
Bar4=pch.anno_barplot(df_bar4,legend=True,cmap='turbo'),
legend=True,legend_gap=5,hgap=0.5)
row_ha = pch.HeatmapAnnotation(
Scatter=pch.anno_scatterplot(df_heatmap.sample4.apply(lambda x:round(x,2)),
height=15,cmap='jet',legend=False),
Bar=pch.anno_barplot(df_heatmap.sample4.apply(lambda x:round(x,2)),
height=15,cmap='rainbow',legend=False),
selected=pch.anno_label(df_rows,colors='red',relpos=(-0.05,0.4)),
label_kws={'rotation':15,'horizontalalignment':'left','verticalalignment':'bottom'},
axis=0,verbose=0)
plt.figure(figsize=(5.5, 8))
cm = pch.ClusterMapPlotter(data=df_heatmap, top_annotation=col_ha,right_annotation=row_ha,
col_cluster=True,row_cluster=True,
col_split=df.AB,row_split=2,
col_split_gap=0.5,row_split_gap=0.8,
label='values',row_dendrogram=True,
show_rownames=False,show_colnames=True,
tree_kws={'row_cmap': 'Set1'},verbose=0,legend_gap=5,
cmap='RdYlBu_r',xticklabels_kws={'labelrotation':-90,'labelcolor':'blue'})
plt.savefig("example0.pdf", bbox_inches='tight')
plt.show()
Starting plotting HeatmapAnnotations
Collecting annotation legends..
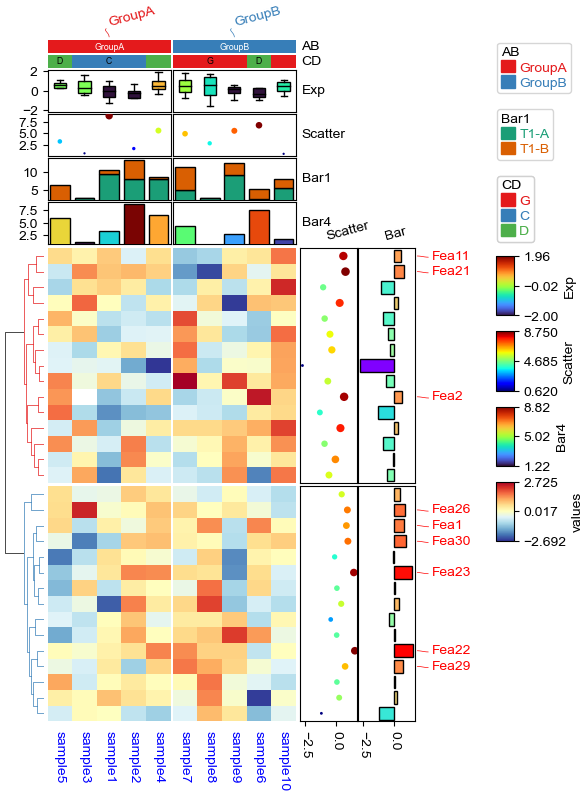